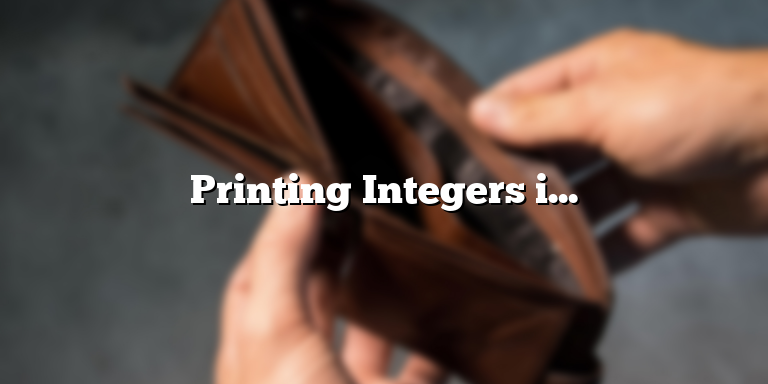
Understanding Integer Printing in C
C is a popular programming language that is used for a wide range of applications. When working with C, one of the key tasks that you will need to carry out is printing integers. Printing integers is essential in programming as it helps to display numerical values in the console or output window. In C, there are several ways to print integers, each with its strengths and weaknesses. In this article, we explore how to print an int in C.
Using printf() Function to Print an int in C
One of the most popular functions used to print integers in C is printf(). This function is easy to use and has a simple syntax. To use the printf() function, you first need to specify a format string that indicates the type of data you wish to print. Then you pass the variable you want to print as an argument to the function. Here is an example:
#include <stdio.h>
int main() {
int num = 42;
printf("The value of num is %d\n", num);
return 0;
}
Here, we declare an integer variable num and assign it a value of 42. We then use the printf() function to print the value of num by passing it as an argument to the function. The format specifier %d is used to specify that we want to print an integer value.
One advantage of using printf() to print integers in C is that it allows you to control the appearance of the output. For example, you can specify the number of digits to display, the width of the output, and whether to left or right justify the output. This makes printf() a powerful tool for formatting output in C.
Other Ways to Print Integers in C
Aside from printf(), there are other functions and methods that you can use to print integers in C. Here are some examples:
- Using puts() Function: The puts() function is mainly used to print strings in C. However, you can also use it to print integers by converting them to strings using sprintf() function. Here is an example:
- Using putchar() Function: The putchar() function is used to print a single character in C. However, you can use it to print the digits of an integer by converting each digit to its corresponding ASCII code. Here is an example:
- Using Puts() Function: Puts() function is a popular function used to print strings in C. But to print an integer using puts() function, one has to convert it into ascii.
#include <stdio.h>
int main() {
int num = 42;
char buffer[10];
sprintf(buffer, "%d", num);
puts(buffer);
return 0;
}
Here, we declare a character buffer called buffer, which we use to store the string representation of our integer value using the sprintf() function. We then print the value of buffer using the puts() function.
#include <stdio.h>
void print_num(int num) {
if (num < 0) {
putchar('-');
num = -num;
}
if (num / 10) {
print_num(num / 10);
}
putchar(num % 10 + '0');
}
int main() {
int num = 42;
print_num(num);
return 0;
}
In this example, we define a recursive function called print_num() that prints the digits of an integer from left to right. We first handle negative numbers by printing a minus sign and then converting the number to a positive value. We then use recursion to print each digit of the number until there are no more digits left.
#include <stdio.h>
int main() {
int num = 23;
char str_num[20];
sprintf(str_num, "%d", num);
puts(str_num);
return 0;
}
We use the sprintf() to convert the integer value to string format, and then theputs() function is used to print the integer to the console or output window.
Conclusion
When it comes to printing integers in C, there are several methods you can use. Each method has its strengths and weaknesses, so it’s important to choose the right one for your particular use case. If you need to format the output, then the printf() function is a good choice. If you want to print each digit of the number, then the putchar() function can be used. I hope this article has helped you understand how to print an int in C.
How to Print an Int in C
Method One: printf
One of the most common ways to print an integer in C is using the printf function. The printf function allows you to output a formatted string to the console, with the values of certain variables filled in. To print an integer using printf, you simply need to include the %d format specifier in your format string, followed by the actual integer that you want to print.
Here’s an example:
// declare and initialize an integer variable
int num = 42;
// use printf to print the integer to the console
printf("The value of num is: %d", num);
The above code will output the following to the console:
The value of num is: 42
As you can see, the %d format specifier is replaced by the value of the num variable when the printf function is executed.
You can also use additional formatting options with printf, such as specifying the minimum width and precision of the printed value, as well as adding leading zeros or a plus sign to positive numbers. Here’s an example:
// declare and initialize an integer variable
int num = 42;
// use printf with formatting options to print the integer to the console
printf("The value of num is: %05d\n", num);
printf("The value of num with a plus sign is: %+d\n", num);
printf("The value of num with precision is: %.3d\n", num);
The above code will output the following to the console:
The value of num is: 00042
The value of num with a plus sign is: +42
The value of num with precision is: 042
As you can see, the formatting options allow you to customize the appearance of the printed integer.
Method Two: putc and putchar
Another way to print an integer in C is using the putc and putchar functions. These functions allow you to output a single character to the console. To print an integer using putc or putchar, you need to convert the integer to a string first, and then output each character of the string using the putc or putchar function.
Here’s an example:
// declare and initialize an integer variable
int num = 42;
// convert the integer to a string
char numString[10];
sprintf(numString, "%d", num);
// output each character of the string to the console using putc
for(int i = 0; i < strlen(numString); i++) {
putc(numString[i], stdout);
}
The above code will output the following to the console:
42
As you can see, the putc function is used to output each character of the numString array to the console.
The putchar function can also be used to achieve the same result, but it only outputs a single character at a time. Here’s an example:
// declare and initialize an integer variable
int num = 42;
// convert the integer to a string
char numString[10];
sprintf(numString, "%d", num);
// output each character of the string to the console using putchar
for(int i = 0; i < strlen(numString); i++) {
putchar(numString[i]);
}
The above code will output the same result as the putc example.
Conclusion
Printing an integer in C is relatively simple using the printf function, and you can customize the appearance of the printed integer using formatting options. If you need to output a single character at a time, you can also use the putc or putchar functions by converting the integer to a string first. Whether you choose to use printf or putc/putchar, both methods are effective ways to output integers to the console.
Method Two: putchar
Another easy way to print integers in C is to use the putchar function. This function outputs a single character to the console, which means you need to convert the integer to a character before printing. Here is an example:
int num = 123; char digit; while (num > 0) { digit = num % 10 + '0'; putchar(digit); num /= 10; }
This code starts with an integer variable num containing the number to be printed. The digit variable is used to hold each digit as it is printed, converted to a character using the ASCII code for ‘0’ (which is 48). The while loop iterates while num is greater than zero, extracting each digit from num and printing it with putchar().
The putchar() function can also be used to output individual characters without converting them. Here is an example that shows how you can use putchar() to print integers directly:
int num = 123; putchar(num / 100 + '0'); putchar(num / 10 % 10 + '0'); putchar(num % 10 + '0');
This code uses integer division and the modulus operator to extract each digit of the number, add it to the ASCII code for ‘0’, and output it to the console with putchar(). By doing this for each digit, we get a full representation of the number.
In conclusion, the putchar() function is a simple and effective way to print integers in C. It requires a little bit of extra work to convert the digits to characters, but this is a small price to pay for the flexibility and control it provides. Try it out in your own code and see how it can make your printing more efficient and readable!
Formatting Integers
Printing integers is one of the most common operations in programming and C language provides us with a number of ways to do it. In this article, we will explore how to print integers in different formats using C.
Printing integers in decimal format
The decimal format is the most basic and commonly used format for printing integers. We can print an integer in decimal format using the `%d` format specifier. Consider the following code snippet:
“`
int num = 42;
printf(“The number is %d”, num);
“`
In the above snippet, we are printing the integer value of `num` using the `%d` format specifier. The output of the above code snippet will be:
“`
The number is 42
“`
Printing integers in hexadecimal format
Hexadecimal is a base-16 numbering system, which is commonly used in computer programming. In C, we can print an integer in hexadecimal format using the `%x` format specifier. Consider the following code snippet:
“`
int num = 255;
printf(“The hex value of num is %x”, num);
“`
In the above snippet, we are printing the hexadecimal value of `num` using the `%x` format specifier. The output of the above code snippet will be:
“`
The hex value of num is ff
“`
Additionally, we can use the `%X` format specifier to print the hexadecimal value in uppercase letters.
Printing integers in octal format
Octal is a base-8 numbering system, which is also commonly used in computer programming. In C, we can print an integer in octal format using the `%o` format specifier. Consider the following code snippet:
“`
int num = 63;
printf(“The octal value of num is %o”, num);
“`
In the above snippet, we are printing the octal value of `num` using the `%o` format specifier. The output of the above code snippet will be:
“`
The octal value of num is 77
“`
Printing integers with width and precision
In C, we can specify the width and precision of the printed integer using the `%[width].[precision]d` format specifier. The width specifies the minimum number of characters to be printed, while the precision specifies the maximum number of digits after the decimal point. Consider the following code snippet:
“`
int num = 123;
printf(“The number is %5.2d”, num);
“`
In the above snippet, we are printing the value of `num` with a width of 5 and a precision of 2 using the `%5.2d` format specifier. The output of the above code snippet will be:
“`
The number is 00123
“`
Printing integers with leading zeros
In some applications, we may want to print integers with leading zeros. In C, we can achieve this by using the `%0[width]d` format specifier. The `0` before the width specifies that the padding should be with zeros instead of spaces. Consider the following code snippet:
“`
int num = 42;
printf(“The padded number is %05d”, num);
“`
In the above snippet, we are printing the value of `num` with a width of 5 and leading zeros using the `%05d` format specifier. The output of the above code snippet will be:
“`
The padded number is 00042
“`
Conclusion
Printing integers in C is a basic yet essential task in programming. C language provides us with a range of format specifiers that we can use to print integers in different formats. By understanding these format specifiers, we can print integers with the desired precision, width, padding, and formatting.
Printing Integers using printf and putchar
Printing integers in C is a simple and basic task. It can be done in different ways, but the most common method is using the printf function. This function is part of the standard output library in C and is used to display formatted output on the screen.
To print an integer using printf, we use the %d format specifier. This specifier tells the printf function to interpret the argument as an integer and display it in decimal format.
Here is an example:
#include <stdio.h>
int main()
{
int num = 5;
printf("The integer is: %d", num);
return 0;
}
The output of this code will be:
The integer is: 5
Another way to print integers in C is using the putchar function. This function is used to display a single character on the screen. We can use a loop to print each digit of the integer one by one using putchar.
Here is an example:
#include <stdio.h>
int main()
{
int num = 5;
int digit;
while (num != 0)
{
digit = num % 10; // get the rightmost digit
putchar(digit + '0'); // display the digit
num /= 10; // remove the rightmost digit
}
return 0;
}
The output of this code will be:
5
Here, we first get the rightmost digit of the integer using the modulo operator and print it using putchar. We then remove this digit from the integer by dividing it by 10. We repeat this process until there are no more digits left to print.
Printing Integers in Specific Formats
We can use different format specifiers to display integers in different formats. Here are some examples:
#include <stdio.h>
int main()
{
int num = 5;
printf("Decimal: %d\n", num);
printf("Octal: %o\n", num);
printf("Hexadecimal: %x\n", num);
printf("Unsigned: %u\n", num);
printf("Exponential: %e\n", num);
printf("Floating point: %f\n", num);
return 0;
}
The output of this code will be:
Decimal: 5
Octal: 5
Hexadecimal: 5
Unsigned: 5
Exponential: 5.000000e+00
Floating point: 5.000000
Here, we use different format specifiers to display the integer in decimal, octal, hexadecimal, unsigned, exponential, and floating-point formats.
Conclusion
Printing integers in C is a basic task, and it can be done using printf and putchar functions. Format specifiers can be used to display integers in different formats. It is essential to understand the different format specifiers to display integers correctly.
By following the examples discussed in this article, you should now be able to print integers in C in different formats.